Introduction
Entity Framework Core commands are useful and make our life a bit easy to execute a few tasks. EF Core is popular Entity Framework data access technology. Entity Framework (EF) Core is a lightweight, extensible and open source. EF Core can serve as an object-relational mapper (O/RM) and it supports many database engines.

I will cover all the options from the three commands.
Commands:
- database Commands to manage the database.
- dbcontext Commands to manage DbContext types.
- migrations Commands to manage migrations.
Short video tutorial
Installation
.NET Core CLI
Use the following .NET Core CLI command from the operating system's command line to install or update the EF Core SQL Server provider
dotnet add package Microsoft.EntityFrameworkCore.SqlServer
Visual Studio NuGet Package Manager Console
Install-Package Microsoft.EntityFrameworkCore.SqlServer
Get the .NET Core CLI tools
dotnet ef
must be installed as a global or local tool.
Also, Install the latest Microsoft.EntityFrameworkCore.Design
package
dotnet tool install --global dotnet-ef dotnet add package Microsoft.EntityFrameworkCore.Design
Refer to how to install Entity Framework Core for more information.
Demo App Explained
For the purpose of the demo, I have a sample restaurant app created using DOTNET CORE. Using the app, we will learn how to use all the dotnet ef commands.
Project structure:
The sample app project has five projects in it. Each serves its purpose and it satisfies the separation of concerns. In the real world, the project has more than one layer hence I choose to demo in a similar structure.
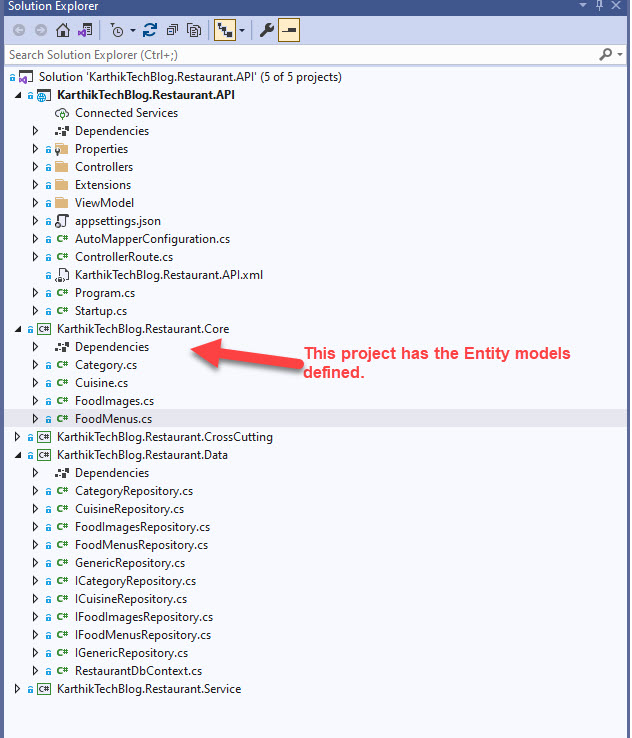
Entity Classes
FoodMenus { get; set; } } public class FoodImages { public int Id { get; set; } public byte[] Image { get; set; } public string Mime { get; set; } public string ImageName { get; set; } public bool IsActive { get; set; } public int? FoodMenusId { get; set; } public virtual FoodMenus FoodMenus { get; set; } } public class FoodMenus { public int Id { get; set; } [Required] [MinLength(5), MaxLength(100)] public string Name { get; set; } [Required] [MinLength(100), MaxLength(5000)] public string Description { get; set; } public decimal Price { get; set; } public bool IsActive { get; set; } public short? CategoryId { get; set; } public virtual Category Category { get; set; } public int? CuisineId { get; set; } public virtual Cuisine Cuisine { get; set; } public virtual ListFoodImages { get; set; } }
Now, Let's get started in exploring each command from dotnet ef command.
Using .NET CORE CLI : Command Prompt
- I have opened the database layer project's location in the command prompt. E.g. "KarthikTechBlog.Restaurant.Data".
2. To check entity framework core is installed and ready to use, type "dotnet ef" in the command prompt and you will see similar or same details as shown in the image.

EF Core Commands in Action
migrations : add
dotnet ef migrations add InitialCreate -s ../KarthikTechBlog.Restaurant.API/KarthikTechBlog.Restaurant.API.csproj
To create migrations from the application, we use the command migration add followed by name of the migration. -s "location of the startup project" is to specify the startup project. This is required for the project to build and generate the migration.

To generate SQL script for the Entity models, use the below command. Remember the script can be generated only when migrations are created.
migrations : script
dotnet ef migrations script -s ../KarthikTechBlog.Restaurant.API/KarthikTechBlog.Restaurant.API.csproj

migrations: list
This lists all the migrations present in the project.
dotnet ef migrations list -s ../KarthikTechBlog.Restaurant.API/KarthikTechBlog.Restaurant.API.csproj
migrations: remove
Rollbacks the latest migration.
dotnet ef migrations remove-s ../KarthikTechBlog.Restaurant.API/KarthikTechBlog.Restaurant.API.csproj
database: bundle
Creates an executable to update the database.
dotnet ef migrations bundle -s ../KarthikTechBlog.Restaurant.API/KarthikTechBlog.Restaurant.API.csproj
database : update
This command updates the database with the details present in the migration. If the database is not present, it will create one for us.
dotnet ef database update -s ../KarthikTechBlog.Restaurant.API/KarthikTechBlog.Restaurant.API.csproj
database: drop
Deletes the database. some of the options are
--force
or-f
which is used to just delete without confirmation.--dry-run
option show which database would be dropped, but don't drop it.
dotnet ef database drop
dbcontext: scaffold
Generates code for a DbContext
and entity types for a database. In order for this command to generate an entity type, the database table must have a primary key.
To scaffold all schemas and tables and puts the new files in the Models folder.
dotnet ef dbcontext scaffold "Server=(localdb)\mssqllocaldb;Database=Shopping;Trusted_Connection=True;" Microsoft.EntityFrameworkCore.SqlServer -o Models
For more options, visit MSDN
dbcontext: optimize
Generates a compiled version of the model used by the DbContext
. Added in EF Core 6.
dotnet ef dbcontext optimize
dbcontext: script
Generates a SQL script from the DbContext. Bypasses any migrations.
dotnet ef dbcontext script
Summary
This post covers Entity Framework Core commands and their usage with examples. I hope you enjoyed and learned something new today.