In this post, I will show you how destructuring JavaScript objects and deep assignment works in JavaScript. This is the continuation of my previous post Destructuring javascript array and its different usages.
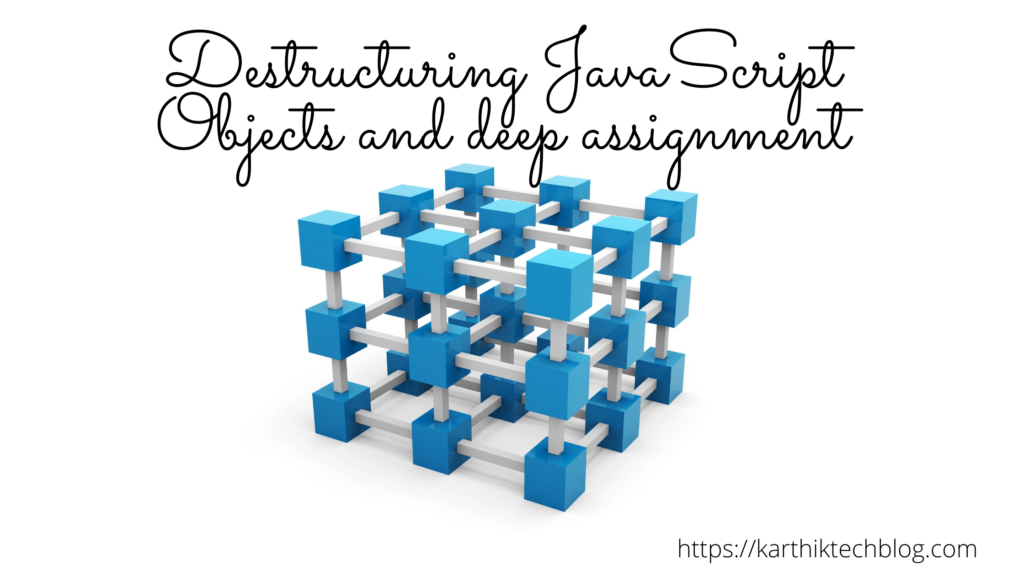
Destructuring JavaScript objects is very useful. I can use it for a deep object structure as well.
In this example, I wanted to assign properties returned from an object to each individual variables.
var getPersonProfile = function() { return { firstName: "Karthik", lastName: "K", twitter: "KarthikTechBlog" } } var objectAssignmentExample = function() { let { firstName: firstName, twitter: twitter } = getPersonProfile(); console.log(firstName); // this produce "Karthik" console.log(twitter); // this produce "KarthikTechBlog" }
This looks like I'm building an object but that is not the case. However, I'm building an object literal as the return value from the calling function is an object. Note that I have used curly brace "{", "}" as it is an object.

In other words, I'm telling JavaScript to assign firstName from the object returned from the getPersonProfile function. his way, firstName property in the objects are assigned to variable name firstName and similarly for twitter variable.
The right-hand side of the colon that is defining my variable. Properties that match the variable name will have the value assigned to it.
var getPersonProfileWithShortName = function() { return { first: "Karthik", lastName: "K", twit: "KarthikTechBlog" } } var objectAssignmentExample1 = function() { let { first: firstName, twit: twitter } = getPersonProfileWithShortName(); console.log(firstName); // this produce "Karthik" console.log(twitter); // this produce "KarthikTechBlog" }
In this example, property name first matches and assigned value to firstName variable.
Deep Object Assignment
In this example, twitter property is inside social property. To access twitter property, you need to navigate to the social to get its value.
var getPersonProfile = function() { return { firstName: "Karthik", lastName: "K", social: { twitter: "KarthikTechBlog" } } } var objectAssignmentExample = function() { let { firstName: firstName, social: { twitter : twitter } } = getPersonProfile(); console.log(firstName); // this produce "Karthik" console.log(twitter); // this produce "KarthikTechBlog" }
Conclusion
In this post, I showed how destructuring JavaScript objects and deep object assignment works. If you have any questions or just want to chat with me, feel free to leave a comment below.