One of the new features in ES6 is the ability to destructing assignment. Destructing is an operation that you might have seen in other languages like Python or Ruby.
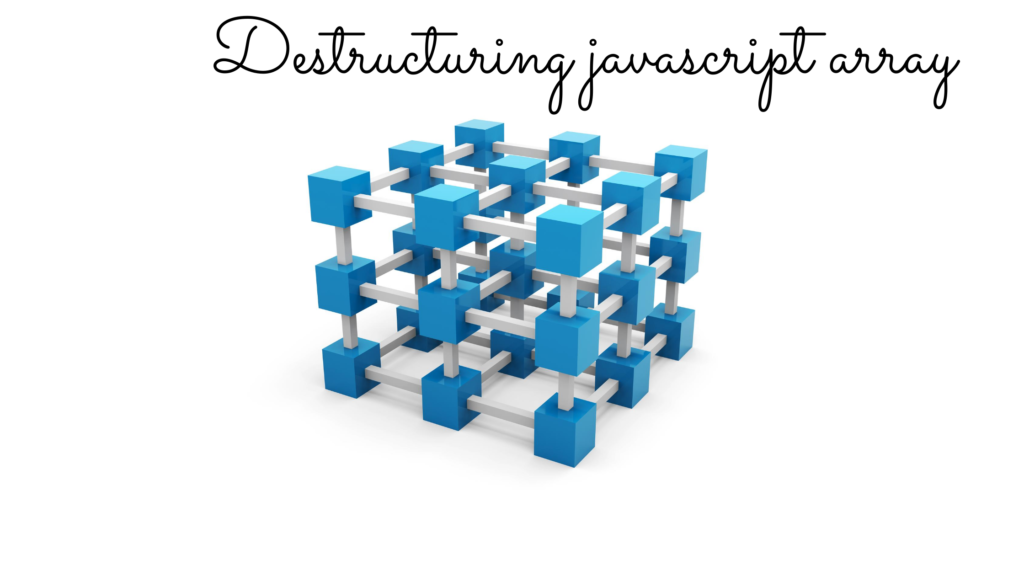
Destructuring operation allows us to assign value to a set of variables by destructing it and doing pattern matching.
A complex data structure like an array or an object full of properties is a good example.
Let's take an example. Two variables X and Y initialized with 2 and 3 values. In the third line we use destructing assignment to say give the value X and Y to Y and X. This will change the value of X to be 3 and Y to be 2 without using any other variable to swap its value.
Destructuring JavaScript Array
var destructingVariables = function () { let x = 2; let y = 3; [x, y] = [y, x]; }
It is important to understand that what we see in the right hand side of this assignment is the array built with the values in Y and X.
To the left-hand side, it is not an array. It looks like I'm building an array literal. However, I'm working with individual variables X and Y and I'm surrounding them with square brackets. This is because I'm destructuring an array.
In simple form, I'm telling JavaScript to take the first item in the array and put it into X and the second one into Y.
var example1 = function () { let [x, y] = [2, 3]; }
I can simplify this by mentioning let there be two variables X and Y.
var example2 = function () { let [x, y] = returnTwoNumbers(); } var returnTwoNumbers = function() { return [2, 3]; }
I can even call an function and assign the values returned from it.
What if the function returns more than expected values? I can still use it by this way.
var returnThreeNumbers = function() { return [4, 2, 3]; } var example3 = function () { let [, x, y] = returnThreeNumbers(); }
Output for all these examples are still same.
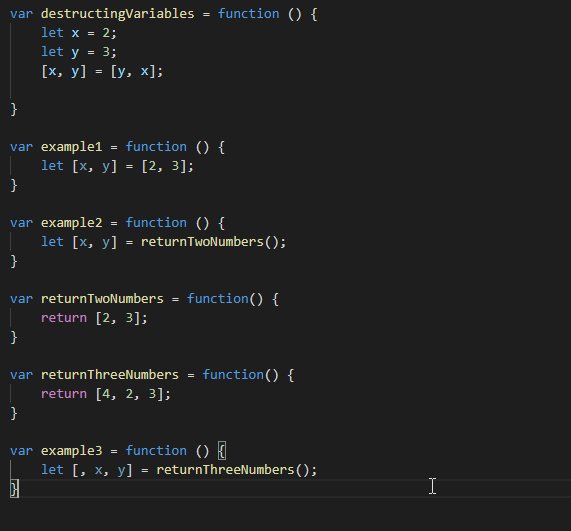
var returnThreeNumbers = function() { return [4, 2, 3]; } var example3 = function () { let [, x, y, z] = returnThreeNumbers(); if(z===undefined) { alert('z is undefined'); } }
If I am trying to assign value to variable Z then Z will be undefined as there is no fourth value from the calling function.
Reference Post
Conclusion
In this post, I explained the destructuring javascript array and its different usages. I also showed how typescript shows the error upfront in visual studio code IDE. If you have any questions or just want to chat with me, feel free to leave a comment below.