In this post, I will explain the difference between var, let, and const in Javascript.

I will be using a visual studio code for the demo purpose. This module is part of variables and parameters which is part of JavaScript Fundamentals for ES6.
These features are designed to make JavaScript code easier to read, write, and also to make this code safer. This will help avoid some dangerous behavior in javascript.
There are two new keywords that are used in declaring variables such as let, const, and also I will explain the difference with the var keyword.
var and let Keyword
let keyword allow us to define variable in JavaScript. var keyword can also be used to define variable. However, var keyword has some limitation when it comes to scope.
what is scope then? The scope of the variable is the area of the program where it is legal to use. var has only two scopes such as Global Scope and Function Scope. There is no block scope for var keyword.
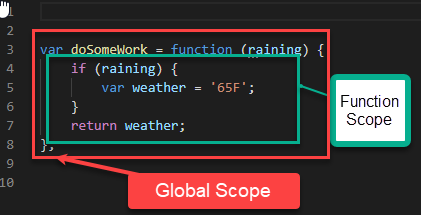
// var Keyword scope example var doSomeWork = function (raining) { if (raining) { var weather = '65F'; } return weather; };
let keyword solve this problem.
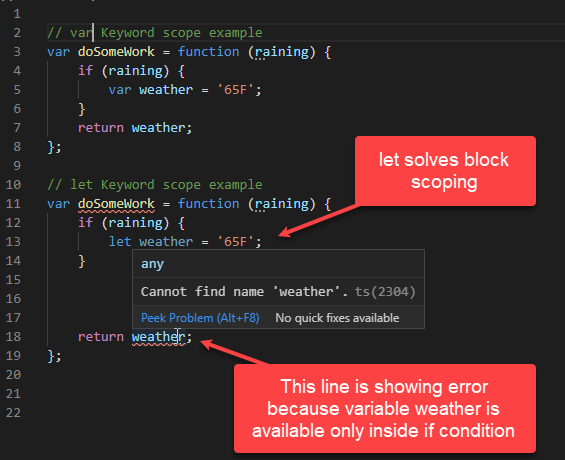
// var Keyword scope example var doSomeWork = function (raining) { if (raining) { var weather = '65F'; } return weather; }; // let Keyword scope example var doSomeWork = function (raining) { if (raining) { let weather = '65F'; return weather; } return '85F'; };
since variable "x" is available throughout the function there is no error. let the keyword makes the variable scoped to the condition and hence we got an error. Using such code through javascript runtime error stating no such variable exist.
All these confusion with the var keyword is because of the Hoisting. Hoisting is JavaScript's default behavior of moving declarations to the top. You can read more here.
Another example is to use let keyword in for loops.

const Keyword
The const keyword is another addition in ECMA Script 6 (ES6). The idea of using this is to create and initialize a read only variable. A variable that hold a constant value something that you never change.
ES6 for const will have block scoping.
var constFuntion = function () { const max_size = 20; max_size = 20 + 20; // syntax error as you cannot assign value again; }

Another difference with const and var keyword is you can define an variable more than once with var keyword. However, you cannot do the same with const keyword.

Conclusion
In this post, I showed the difference between var, let, and const in Javascript. I also showed how typescript shows the error upfront in visual studio code IDE. If you have any questions or just want to chat with me, feel free to leave a comment below.