Introduction
In this JavaScript Getting Started, you will learn about JavaScript Variables var, let, and const along with their usage. Join me in learning variables and start using them in your work.
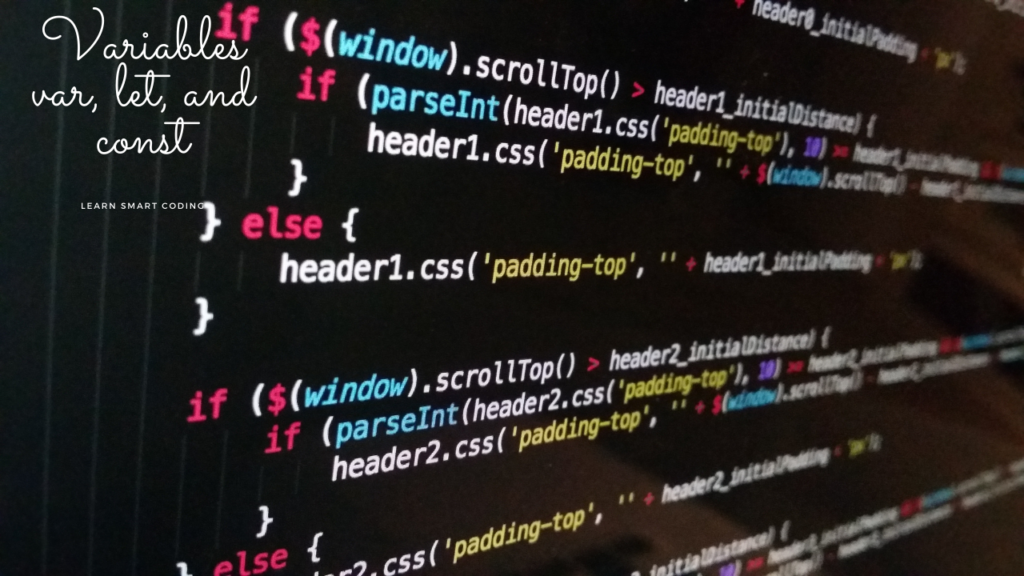
What is a variable ?
Any application that we write is going to be based on data. If we're selling fruits, we need data on the fruit name, the price, and all the data that belongs to an invoice or a purchase order. Likewise, if you consider your work is around car company then you need all car-related information such as engine, model, price, etc. I can talk about many such examples. All deals with data.
In JavaScript and most other programming languages, we use variables to hold this information. Data is stored in the computer memory. Let's take an example. If you had to sell a car which is of price $20,000
.
That needs to be stored somewhere in the computer's memory and typically, a memory address is a long, complicated number. It would be very difficult if we had to use this long number to reference that value.
So instead we use a variable. In this case, we can use a variable called carPrice
. So in this case we were using an English word to represent the number in the computer, and you can use words and characters from your own native language and name it in an understanding way.
There's a special terminology we use in programming when you create a variable. It's called declaring the variable.
Declaring Variables
We declare variables to let Javascript know that we are going to work with variables. When we declare a variable, we use a keyword, let. A keyword is a special symbol that JavaScript knows about.
It performs some type of action. In this case, let allows us to declare a variable, and after the keyword let, we have the variable name that we want to use and there are certain rules for creating variable names. we will talk about the variable naming shortly.
Keywords let and var
We have been using the var keyword in JavaScript. However, the var keyword has some limitations when it comes to scope. There are only two scopes for var which are the global scope and function scope. There is no block scope. This often becomes a bug for many developers.
what is scope then? The scope of the variable is the area of the program where it is legal to use. var has only two scopes as Global Scope and Function Scope. There is no block scope for var keyword.
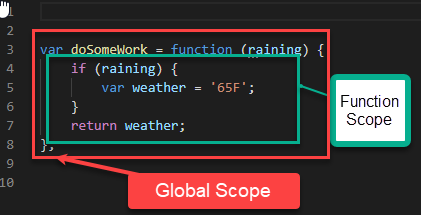
// var Keyword scope example var doSomeWork = function (raining) { if (raining) { var weather = '65F'; } return weather; };
let keyword solve this problem.
let is a new Keyword in JavaScript and allows us to define variables.
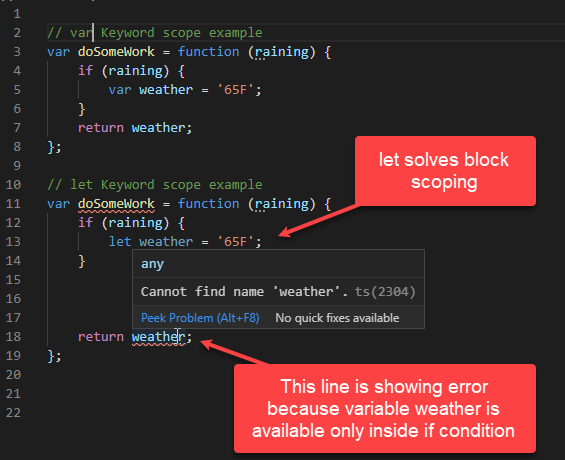
// var Keyword scope example var doSomeWork = function (raining) { if (raining) { var weather = '65F'; } return weather; }; // let Keyword scope example var doSomeWork = function (raining) { if (raining) { let weather = '65F'; return weather; } return '85F'; };
since variable "x" is available throughout the function there is no error. let the keyword makes the variable scoped to the condition and hence we got an error. Using such code through javascript runtime error stating no such variable exist.
All this confusion with the var keyword is because of the Hoisting. Hoisting is JavaScript's default behavior of moving declarations to the top. You can read more here.
Another example is to use the let keyword in for loops.

Variable naming
- The name must contain only letters, digits, or the symbols
$
and_
- The first character must not be a digit.
Try to provide a meaningful name and limit the length of the variable to a max of 15 characters.
example for variable naming
let $ = 20; let _ = "John" let _name$ = "name"
const Keyword
The const keyword is another addition in ECMA Script 6 (ES6). The idea of using this is to create and initialize a read-only variable. A variable that holds a constant value is something that you never change.
ES6 for const will have block scoping.
var constFuntion = function () { const max_size = 20; max_size = 20 + 20; // syntax error as you cannot assign value again; }

Another difference with const and var keywords is you can define a variable more than once with the var keyword. However, you cannot do the same with the const keyword.

JavaScript Getting Started: Table of contents
- Introduction and Setting up a local environment
- Variables and constants
- Types and Operators
- Program flow : conditions, comparision and different loops.
- Parameters : Default, Rest ...Spread and templates
- Functions
- Objects and the DOM
- Arrarys
- Scope and Hoistings
- Classes (Advance)
Summary
This post JavaScript Getting Started covers Variables var, let, and const, how we declare variables, and the usage of var, let, and const keywords.